Control Library
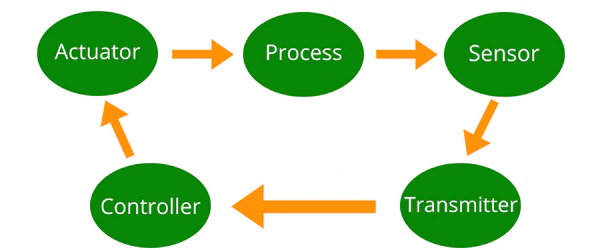
Concept
Implement commonly used algorithms used in control loops. This typically covers PID control loops and their supporting elements like filters, scaling, glitch detection and similar.
Why
Control loops typically interacts with the real world. The real world is complex enough, without additional layers of code complexity. The goal of the project is to keep the implementation clear and easy to understand. Code that is easy to understand becomes easy to maintain.
How
Implement in C++ taking advantage of object orientated features to present a clean API for the user. Should not rely on integer math and optimization. Floats and integers both have their pros and cons. Floating Point are avaible on most micro controllers and the user should not worry about premature optimization. Slow routines may be updated at a later time if the need arises.
The library should remain cross-platform to ensure the code may be used for embedded development and on the desktop. All the way from 8-bit Arduino to 64-bit Windows.
Try to let the final implementation reflect control loop diagrams as closely as possible.
TDD
Unit test individual components to ensure their reliability before integration. Compare with other implementations for correcteness taking into account floating point (in)accuracy.
Features
- PID controller
- P/PI/PID
- Various clamping/anti-windup strategies.
- Filters
- Exponentional Moving Average
- Rolling average
- Kalman Filter
- FIR/IIR
- Detection
- Inside/Outside range, inhibit time, hysteresis, glitch protection
- Deadbands
- Ramp / Fade
- Expontential, Linear
- Generators
- Sine, Square, Triangle, Sawtooth
- Utils
- Linear scaling (Y=ax+b)
- Polyominal
- Map
- Limit
Example
graph LR;
Input-->Map-->Filter-->Error-->PID-->Limit-->Output;